Class Loader Subsystem
The main function of class loader subsystem is to load .class files to the various memory areas of JVM. The class loader subsystem internally contains three main activities.
1-Loading
2-Linking
3-Initialization
Fig I:
The class ClassLoader is an abstract class that is responsible for loading classes.
1-Loading
This is the first activity of class loader subsystem.The loading activity loads .class(binary) files into the method area with the various information contains by its (.class) file.What type of informations store by JVM in method area is given below.
2-Linking
After loading .class files into method area JVM will creates seperate class class object to know the class level information and stored into heap memory area.The programmer and also JVM use this class class object to get all the class level information.
In linking activity comprises three other activities like verification, prepration, and resolution.
I-Verification
II-Preparation
III-Resolution
Before going to discuss in details I will explain what is a classclass object and how programmers can use this. Below is the demo example for how to use class-class object and how to implement in programming practice to get all class level information like all methods and fields present inside a class.
Demo Example:
import java.lang.reflect.*;
class Employer {
private String name;
private double salary;
public String getName() {
return name;
}public void setEmployerSalary(double salary) {
this.salary = salary;
}
}
class ClassClassObjectDemo{public static void main(String[] args) throws ClassNotFoundException{
Class obj = Class.forName(?Employer?);
// This statement load the Employer .class file and return class
// class
// object.Method[] m = obj.getDeclaredMethods();
for(Method m1:m){
System.out.println(m1);
}Field[] f = obj.getDeclaredFields();
for(Field f1:f) {
System.out.println(f1);
}}
}
Save the above source file as ClassClassObjectDemo.java
Run the program using cmd :
In my previous tutorials, we will discuss how to run java programs using cmd. Open a command prompt and type the below commands.
C:UsersMangalamDigital>D:
D:>javac ClassClassObjectDemo.java // My java source file stored location
D:>java ClassClassObjectDemo
public java.lang.String Employer.getName()
public void Employer.setEmployerSalary(double)
private java.lang.String Employer.name
private double Employer.salary
I-Verification
After loading .class files into method area JVM always checks the converted byte code are properly formatted as per java language. Byte code verifier is responsible to perform this activity. So this is the main reason Java is secure and very robust language. If JVM detects the loaded .class file is not well formatted or any sort of security problem then immediately throws VerifyError.This is the child class of LinkageError which reside inside java.lang package. After performing this activity the next activity is preparation.
II-Preparation
After performing verification activity the JVM allocates memory area for class level static variable and assigned default value just like below.
Example:
boolean b=false;
int b=0;
double b=0.0;
III-Resolution
For every symbol, JVM creates a separate constant pool for storing symbolic references used in a class. Just for understanding take a Demo class which is converted ( .class ) file named with Demo.class and stored into method area.
3-Initialization
In initialization activity, the default value assigned to the static variable replaced with the actual values and static blocks are executed from top to bottom line by line.
Fig.2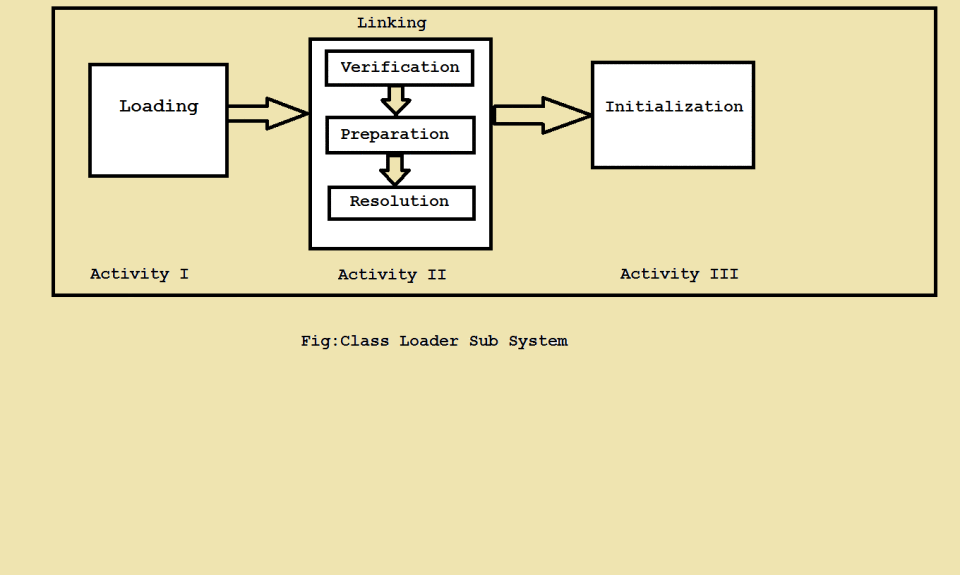
Fig.3
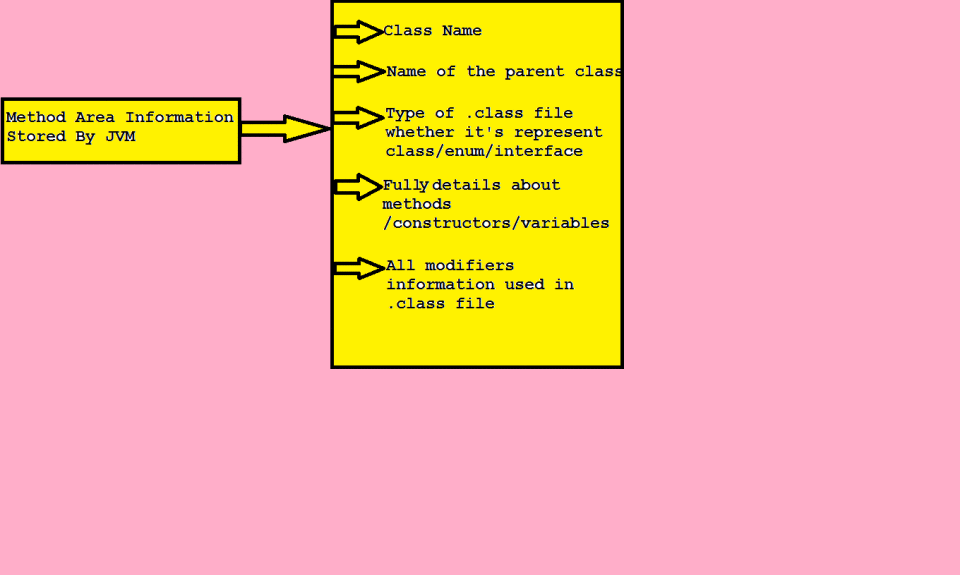