Top Python & ML Interview Questions
Interview Questions
Q1- Detect loop in the linked list (figure1)
Ans-
Sample code
class BaseNode:
def __init__(self, data):
self.data = data
self.next = None
class List:
# Function to initialize head
def __init__(self):
self.head = None
def push(self, new_data):
new_node = BaseNode(new_data)
new_node.next = self.head
self.head = new_node
def printList(self):
temp = self.head
while(temp):
print (temp.data, end =" ")
temp = temp.next
def detect_loop(self):
s = set()
temp = self.head
while (temp):
if (temp in s):
return True
s.add(temp)
temp = temp.next
return False
list =List()
list.push(1)
list.push(2)
list.push(3)
list.push(4)
# Create a loop using
list.head.next.next.next.next = list.head;
if( llist.detect_loop()):
print ("Loop found")
else :
print ("No Loop ")
Q2- Replace the “u” with “at” and “n” with “ur” in the following string sequences.
str1=’sunday’
Ans-
str1='sunday'
str2=str1.replace('u','at').replace('n','ur')
print(str2)
Q3-Describe the term Bias-Variance Tradeoff in Machine Learning
Ans –
Bias, Variance is the main factor that leads to the models are underfitting and overfitting. For the good model, it must be in Low bias and low variance.
Q4-What is pickling and unpickling in python
Ans –
Pickling
The string representation of any object and dumps it into a file is known as pickling.
Sample Code:
import pickle
data1 = {'a': 'test',
'b': ('test'),
'd': None}
output = open('pickle.pkl', 'wb')
pickle.dump(data1, output)
output.close()
Unpickling
While the retrieving of the String representation of the object from file is known as unpickling.
To read from a pickled file
Sample Code:
import pickle
pk_file = open('pickle.pkl', 'rb')
data1 = pickle.load(pk_file)
pk_file.close()
Thank you for reading!
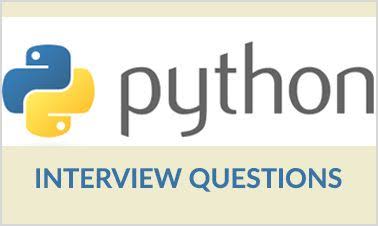